If you are looking for a way to subclass a standard file open dialog from .NET, take a look at the Vista Bridge Sample Library from Microsoft.
„Subclassing“ (or „Extending“, „Customizing“) refers to adding new controls to the bottom of the standard Windows dialog like following, to extend it:
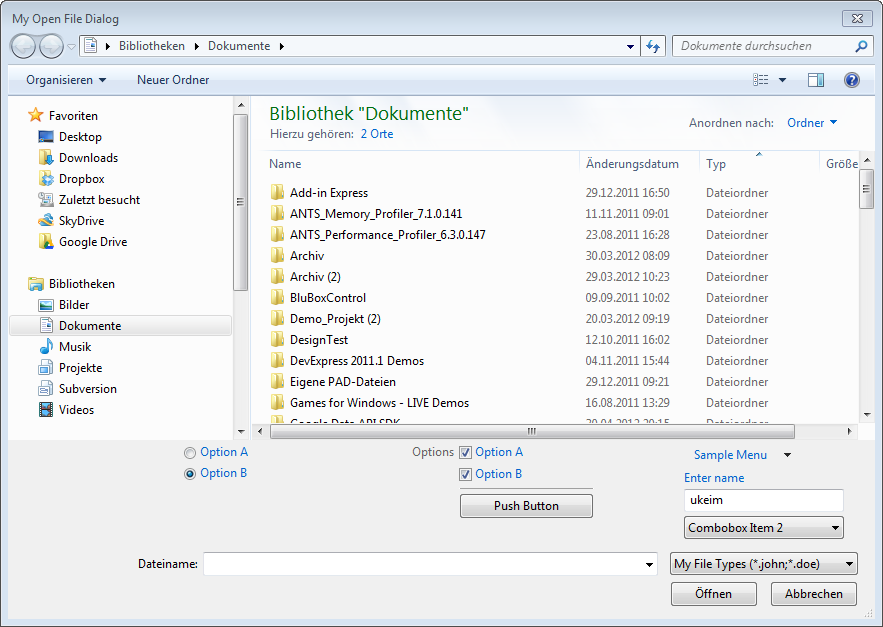
Although the examples are for WPF, I guess it is rather simple to migrate them to WinForms (.NET 2.0).